Hi all,
I created a question using JavaScript to capture three values and the running total per row (each row will be a county the user selects in a previous question). The JavaScript is set to save the embedded data as {County} - the question name - Brief/Intermediate/Extended/Total.
The JavaScript is correctly saving all four entries for each individual response in the Data & Analysis tab. However, when I export the results, there are four columns for each county that are the same value and titled {County} - the question name - Total. The export isn’t saving the Brief, Intermediate, and Extended values the user entered and is overwriting those to save the Total values four times instead of once.
Why are the two sets of embedded data different? And how do I get the data export embedded data to be the same as the embedded data in the individual response?
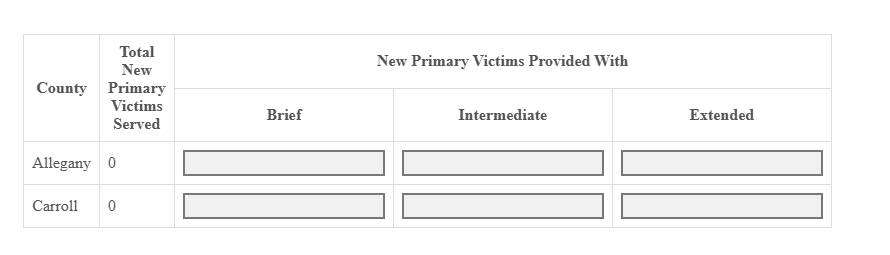
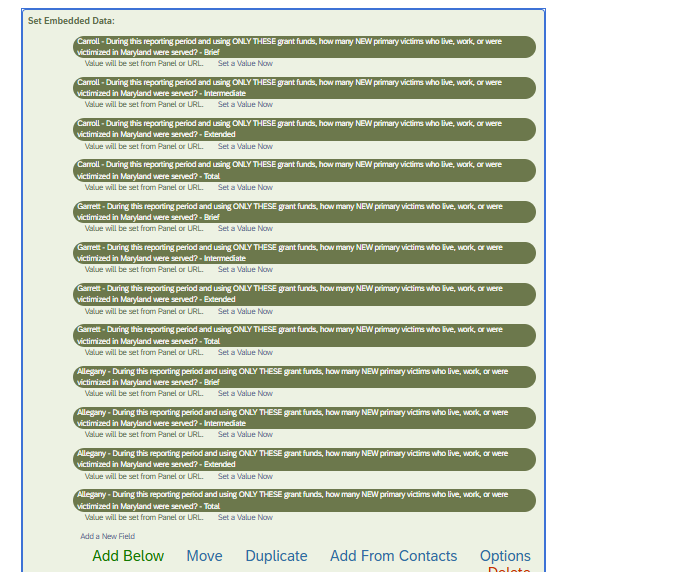
Survey flow embedded data (located at the top of the survey flow):
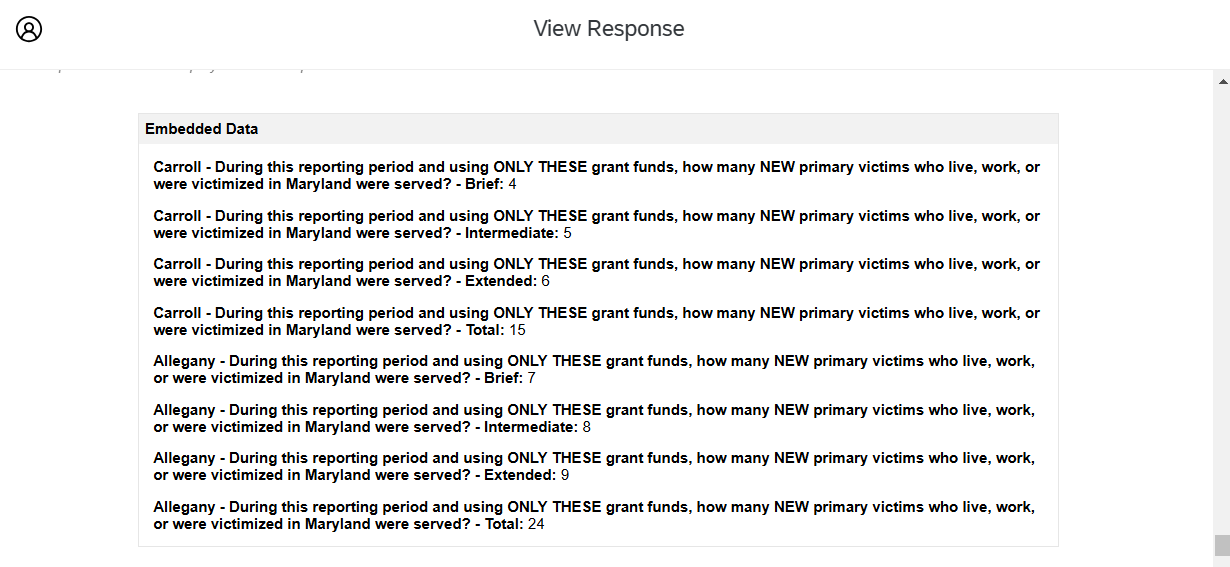

Data export that is only saving totals for each county instead of four values
JavaScript code for QID50:
Qualtrics.SurveyEngine.addOnReady(function() {
console.log("JavaScript Loaded");
// Create the table element
var table = document.createElement('table');
table.id = 'victimTable';
table.style.width = '100%';
table.style.borderCollapse = 'collapse';
// Create table headers
var headerRow1 = table.insertRow();
var headerRow2 = table.insertRow();
var headers = [
{ text: "County", rowspan: 2 },
{ text: "Total New Primary Victims Served", rowspan: 2 },
{ text: "New Primary Victims Provided With", colspan: 3 }
];
headers.forEach(header => {
var cell = headerRow1.insertCell();
cell.textContent = header.text;
cell.style.border = '1px solid #ddd';
cell.style.padding = '8px';
cell.style.fontWeight = 'bold';
cell.style.textAlign = 'center';
if (header.rowspan) cell.rowSpan = header.rowspan;
if (header.colspan) cell.colSpan = header.colspan;
});
// Add columns for each service type (Brief, Intermediate, Extended)
["Brief", "Intermediate", "Extended"].forEach(text => {
var cell = headerRow2.insertCell();
cell.textContent = text;
cell.style.border = '1px solid #ddd';
cell.style.padding = '8px';
cell.style.fontWeight = 'bold';
cell.style.textAlign = 'center';
});
// Function to create a data row for each county
function createDataRow(displayName) {
if (!displayName) return;
console.log("Creating row for:", displayName);
var row = table.insertRow();
// County cell
var countyCell = row.insertCell();
countyCell.textContent = displayName;
countyCell.style.border = '1px solid #ddd';
countyCell.style.padding = '8px';
// Total cell (initialize to 0)
var totalCell = row.insertCell();
totalCell.style.border = '1px solid #ddd';
totalCell.style.padding = '8px';
totalCell.textContent = "0";
row.totalCell = totalCell;
// Store service values for the row
var serviceValues = {
"Brief": 0,
"Intermediate": 0,
"Extended": 0
};
// Service Types (Brief, Intermediate, Extended)
["Brief", "Intermediate", "Extended"].forEach(serviceType => {
var cell = row.insertCell();
cell.style.border = '1px solid #ddd';
cell.style.padding = '8px';
// Create input field for each service type
var input = document.createElement('input');
input.type = 'text';
input.className = 'data-input';
// Unique data index for each input field
input.dataset.index = displayName + " - During this reporting period and using ONLY THESE grant funds, how many NEW primary victims who live, work, or were victimized in Maryland were served? - " + serviceType;
// Load stored value from Embedded Data (if available)
var storedValue = Qualtrics.SurveyEngine.getEmbeddedData(input.dataset.index);
input.value = storedValue !== undefined ? storedValue : ""; // Use undefined check
// Store the initial service value (if stored or set to 0)
serviceValues[serviceType] = parseInt(input.value) || 0;
// Event listener to save input value and update totals
input.addEventListener('input', function() {
this.value = this.value.replace(/[^0-9]/g, ''); // Allow only numeric values
Qualtrics.SurveyEngine.setEmbeddedData(this.dataset.index, this.value);
// Update the service value for the specific service type
serviceValues[serviceType] = parseInt(this.value) || 0;
updateTotal(row, displayName, serviceValues); // Update the total after input
validateInputs();
});
cell.appendChild(input);
});
// Store the county name as Embedded Data
Qualtrics.SurveyEngine.setEmbeddedData(displayName, displayName);
}
// Function to update the total for a county row (after input)
function updateTotal(row, displayName, serviceValues) {
var total = serviceValues["Brief"] + serviceValues["Intermediate"] + serviceValues["Extended"];
// Update the total cell in the row
row.totalCell.textContent = total;
// Store the total in Embedded Data with the specified name format
Qualtrics.SurveyEngine.setEmbeddedData(displayName + " - During this reporting period and using ONLY THESE grant funds, how many NEW primary victims who live, work, or were victimized in Maryland were served? - Total", total);
console.log(displayName + " - Total:", total);
}
// Function to validate that all input fields are filled
function validateInputs() {
var inputs = document.querySelectorAll('.data-input');
let allFilled = true;
let allEmpty = true;
inputs.forEach(function(input) {
if (input.value.trim() === "") {
allFilled = false;
} else {
allEmpty = false;
}
});
// Disable the "Next" button if not all fields are filled or all are empty
var nextButton = document.querySelector('.NextButton');
if (nextButton) nextButton.disabled = allEmpty || !allFilled;
}
// Get selected counties from the survey questions
var selectedCounties = []; // Initialize as an empty array
var primaryChoices = "${q://QID49/ChoiceGroup/SelectedChoices}".trim();
var secondaryChoices = "${q://QID51/ChoiceGroup/SelectedChoices}".trim();
if (primaryChoices) {
primaryChoices.split(",").forEach(county => selectedCounties.push(county.trim()));
}
if (secondaryChoices) {
secondaryChoices.split(",").forEach(county => selectedCounties.push(county.trim()));
}
console.log("Selected Counties:", selectedCounties);
// Create a row for each selected county
selectedCounties.forEach(county => {
createDataRow(county);
});
// Insert the table into the question container (QID50)
var questionContainer = document.getElementById('QID50');
if (questionContainer) {
console.log("QID50 found! Inserting table...");
var questionText = questionContainer.querySelector('.QuestionText');
if (questionText) {
questionContainer.insertBefore(questionText, questionContainer.firstChild);
}
questionContainer.insertBefore(table, questionText.nextSibling);
} else {
console.error("Error: Could not find question container for QID50.");
}
// Function to restore totals on page load
function restoreTotals() {
document.querySelectorAll('tr').forEach(row => {
var county = row.cells[0].textContent;
var serviceValues = {
"Brief": parseInt(row.cells[2].querySelector('input').value) || 0,
"Intermediate": parseInt(row.cells[3].querySelector('input').value) || 0,
"Extended": parseInt(row.cells[4].querySelector('input').value) || 0
};
updateTotal(row, county, serviceValues);
});
}
// Add event listeners for input validation
document.querySelectorAll('.data-input').forEach(input => {
input.addEventListener('input', validateInputs);
});
// Initialize totals and validate inputs on page load
restoreTotals();
validateInputs();
});