A major goal of our recruitment survey is to identify users who are between the ages of 14-24 (target population) and those who are not (not eligible for our study). Currently we ask users for their date of birth (MM/DD/YYYY) and would like to use JS to:
1. Calculate age, based on the current date
2. Determine if the age is within our target range (14-24)
3. Provide a validation alert to the users AND/OR categorize users using embedded data in the categories of eligible/ineligible based on age
Please take a look at the code I have now for the DOB question:
Qualtrics.SurveyEngine.addOnReady(function()
{
var dob_entry = getTextValue();
var split_dob = dob_entry.split("/");
var month = split_dob[0];
var day = split_dob[1];
var year = split_dob[2];
var dob_asdate = new Date(year, month, day);
var today = new Date();
var mili_dif = Math.abs(today.getTime() - dob_asdate.getTime());
var age = (mili_dif / (1000 * 3600 * 24 * 365.25));
within_age_range=(14<age & age<24);
alert(age);
});
Page 1 / 2
The below code is to calculate the age based on the birth year:
Add an embedded data "UserAge" and the below code will assign the age of the user in it. Then in Survey flow, you can add branch logic to categorize user as eligible or ineligible.
!
Add an embedded data "UserAge" and the below code will assign the age of the user in it. Then in Survey flow, you can add branch logic to categorize user as eligible or ineligible.
!
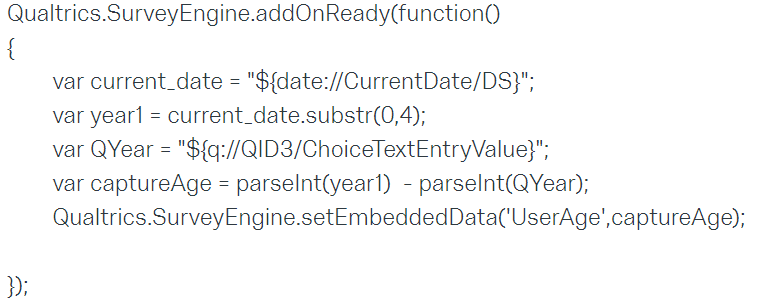
To calculate age based on current DOB you can use the following code:
var dob_entry = getTextValue();
var split_dob = dob_entry.split("/");
var month = split_dob[0];
var day = split_dob[1];
var year = split_dob[2];
var ageValue = 0;
var condition1 = parseInt(month+day);
var today_date = new Date();
var today_year = (today_date.getFullYear()).toString();
var today_day = (today_date.getDate()).toString();
var today_month= (today_date.getMonth()+1).toString();
var condition2 = parseInt(today_month+today_day);
if(condition2>=condition1)
{
ageValue = parseInt(today_year-parseInt(year));
}
else
{
ageValue = parseInt(today_year-parseInt(year)-1);
}
if(14<=ageValue && ageValue<=24)
{ Qualtrics.SurveyEngine.setEmbeddedData('Validity',1);
alert('Valid age.');
}
else
{ Qualtrics.SurveyEngine.setEmbeddedData('Validity',0);
alert('Invalid age.');
}
You'll need to add an embedded data 'Validity' in your Survey Flow and then use the display logic or survey termination condition on embedded data using the set embedded data.
var dob_entry = getTextValue();
var split_dob = dob_entry.split("/");
var month = split_dob[0];
var day = split_dob[1];
var year = split_dob[2];
var ageValue = 0;
var condition1 = parseInt(month+day);
var today_date = new Date();
var today_year = (today_date.getFullYear()).toString();
var today_day = (today_date.getDate()).toString();
var today_month= (today_date.getMonth()+1).toString();
var condition2 = parseInt(today_month+today_day);
if(condition2>=condition1)
{
ageValue = parseInt(today_year-parseInt(year));
}
else
{
ageValue = parseInt(today_year-parseInt(year)-1);
}
if(14<=ageValue && ageValue<=24)
{ Qualtrics.SurveyEngine.setEmbeddedData('Validity',1);
alert('Valid age.');
}
else
{ Qualtrics.SurveyEngine.setEmbeddedData('Validity',0);
alert('Invalid age.');
}
You'll need to add an embedded data 'Validity' in your Survey Flow and then use the display logic or survey termination condition on embedded data using the set embedded data.
Using Mohammedali_Rajapakar_Ugam's method, I can't get the value from the text entry field for QYear to pipe in. I've tried using a form field to ask for the date in YYYY/MM/DD and MM/DD/YYYY formats.
I don't remember seeing this question before @mjs5az resurrected it, but the answers given are doing it the hard way and the accepted answer isn't always accurate. You can do everything @nkumich asked for by using moment.js and adding this script to the DOB question:
```
Qualtrics.SurveyEngine.addOnPageSubmit(function() {
var age = moment().diff(moment(jQuery("#"+this.questionId+" .InputText").val()), 'years');
Qualtrics.SurveyEngine.setEmbeddedData('age', age);
if(age > 13 && age < 25) Qualtrics.SurveyEngine.setEmbeddedData('eligible', '1');
});
```
Demo survey
```
Qualtrics.SurveyEngine.addOnPageSubmit(function() {
var age = moment().diff(moment(jQuery("#"+this.questionId+" .InputText").val()), 'years');
Qualtrics.SurveyEngine.setEmbeddedData('age', age);
if(age > 13 && age < 25) Qualtrics.SurveyEngine.setEmbeddedData('eligible', '1');
});
```
Demo survey
@TomG I'm sorry, I'm not familiar with JS. So, I put this in the question html: !
but I'm not sure how to edit the JS that you provided. Where do I put the QID? Is there anything else that I need to change? I set an "Age" variable in the survey flow. Thanks so much for your help.

I got it to work, I didn't need to change anything. My apologies.
@TomG I want to create a validation message if the age is above > 18. Is there a way to do this without them submitting their response?
> @mjs5zx said:
> @TomG I want to create a validation message if the age is above > 18. Is there a way to do this without them submitting their response?
@mjs5zx - Yes there is, but your exact requirements aren't clear to me (since the original post wasn't yours). Whatever they are, the solution will be more complicated than what I posted.
> @TomG I want to create a validation message if the age is above > 18. Is there a way to do this without them submitting their response?
@mjs5zx - Yes there is, but your exact requirements aren't clear to me (since the original post wasn't yours). Whatever they are, the solution will be more complicated than what I posted.
@TomG Basically, I want it to treat it like the baked in content validation and not allow the participant to proceed with the survey if the age is > 18, and to give them an error message. The birthdate is the first question. We've already collected it in a database application (Filemaker) that is passing some information to Qualtrics. It's not in the process currently, but it would probably be a good idea for me to pass the birthdate from Filemaker and create a flag if it doesn't match the birthdate entered into Qualtrics.
@mjs5zx - You can do age validation through some JavaScript trickery, but I don't think validation is the best choice. I think best practice is to terminate respondents that don't qualify. Otherwise, you are giving them to opportunity (i.e. encouraging them) to lie in order to move forward.
For the birthdate you already have, you can assign it to an embedded variable and pipe that embedded variable into your question as the default answer.
For the birthdate you already have, you can assign it to an embedded variable and pipe that embedded variable into your question as the default answer.
@TomG This works. Thanks so much. Also, I really like how your demo survey automatically adds the '/' to assist with the date format. Would you be willing to help me learn how to do that?
> @mjs5zx said:
> @TomG This works. Thanks so much. Also, I really like how your demo survey automatically adds the '/' to assist with the date format. Would you be willing to help me learn how to do that?
@mjs5zx - I'll send you a message.
> @TomG This works. Thanks so much. Also, I really like how your demo survey automatically adds the '/' to assist with the date format. Would you be willing to help me learn how to do that?
@mjs5zx - I'll send you a message.
> @TomG said:
> > @mjs5zx said:
> > @TomG This works. Thanks so much. Also, I really like how your demo survey automatically adds the '/' to assist with the date format. Would you be willing to help me learn how to do that?
>
> @mjs5zx - I'll send you a message.
@TomG Hi! I would be interesting as well in adding the "/" to assist wit the date format. Would it be possible to get your advice on this? Many thanks!
> > @mjs5zx said:
> > @TomG This works. Thanks so much. Also, I really like how your demo survey automatically adds the '/' to assist with the date format. Would you be willing to help me learn how to do that?
>
> @mjs5zx - I'll send you a message.
@TomG Hi! I would be interesting as well in adding the "/" to assist wit the date format. Would it be possible to get your advice on this? Many thanks!
@TomG In addition, I tried to exclude participants to my survey based on their DOB. I want only people who are between 16 and 99.
1. I've added in Qualtrics header (in Look & Feel) this piece of code: <script type="text/javascript" data-runid="016570089713574543" src="https://cdn.jsdelivr.net/npm/moment@2/moment.min.js"></script>
2. I've added this piece of code in the DOB question:
Qualtrics.SurveyEngine.addOnPageSubmit(function() {
var age = moment().diff(moment(jQuery("#"+this.questionId+" .InputText").val()), 'years');
Qualtrics.SurveyEngine.setEmbeddedData('age', age);
if(age > 15 && age < 100) Qualtrics.SurveyEngine.setEmbeddedData('eligible', '1');
});
3. In the Survey Flow, I've added this:
Branch if eligible is Not Equal to 1 then End of Survey
Am I missing something?
Many thanks for your support!
Best,
Anais
1. I've added in Qualtrics header (in Look & Feel) this piece of code: <script type="text/javascript" data-runid="016570089713574543" src="https://cdn.jsdelivr.net/npm/moment@2/moment.min.js"></script>
2. I've added this piece of code in the DOB question:
Qualtrics.SurveyEngine.addOnPageSubmit(function() {
var age = moment().diff(moment(jQuery("#"+this.questionId+" .InputText").val()), 'years');
Qualtrics.SurveyEngine.setEmbeddedData('age', age);
if(age > 15 && age < 100) Qualtrics.SurveyEngine.setEmbeddedData('eligible', '1');
});
3. In the Survey Flow, I've added this:
Branch if eligible is Not Equal to 1 then End of Survey
Am I missing something?
Many thanks for your support!
Best,
Anais
> @annaj said:
> Am I missing something?
You've got all the correct steps. Your code under (1) doesn't display, but if that is correct, it should work.
> Am I missing something?
You've got all the correct steps. Your code under (1) doesn't display, but if that is correct, it should work.
@TomG Sorry here is the code in (1): !
Is that correct?
I can't understand why it doesn't work. Do I need to add any embedded data?

Is that correct?
I can't understand why it doesn't work. Do I need to add any embedded data?
> @annaj said:
> @TomG Hi! I would be interesting as well in adding the "/" to assist wit the date format. Would it be possible to get your advice on this? Many thanks!
Use cleave.js
> @TomG Hi! I would be interesting as well in adding the "/" to assist wit the date format. Would it be possible to get your advice on this? Many thanks!
Use cleave.js
> @TomG said:
> > @annaj said:
> > @TomG Hi! I would be interesting as well in adding the "/" to assist wit the date format. Would it be possible to get your advice on this? Many thanks!
> Use cleave.js
>
>
@TomG Thanks for the hint! Could you please explain me how to use cleave.js? What piece of code should I add in the question?
> > @annaj said:
> > @TomG Hi! I would be interesting as well in adding the "/" to assist wit the date format. Would it be possible to get your advice on this? Many thanks!
> Use cleave.js
>
>
@TomG Thanks for the hint! Could you please explain me how to use cleave.js? What piece of code should I add in the question?
> @annaj said:
> @TomG Thanks for the hint! Could you please explain me how to use cleave.js? What piece of code should I add in the question?
I've answered this before. Try this thread: https://www.qualtrics.com/community/discussion/comment/2682#Comment_2682
> @TomG Thanks for the hint! Could you please explain me how to use cleave.js? What piece of code should I add in the question?
I've answered this before. Try this thread: https://www.qualtrics.com/community/discussion/comment/2682#Comment_2682
> @TomG said:
> > @annaj said:
> > @TomG Thanks for the hint! Could you please explain me how to use cleave.js? What piece of code should I add in the question?
> I've answered this before. Try this thread: https://www.qualtrics.com/community/discussion/comment/2682#Comment_2682
>
@TomG Many thanks! I will check this out!
> > @annaj said:
> > @TomG Thanks for the hint! Could you please explain me how to use cleave.js? What piece of code should I add in the question?
> I've answered this before. Try this thread: https://www.qualtrics.com/community/discussion/comment/2682#Comment_2682
>
@TomG Many thanks! I will check this out!
@TomG I've aded in a previous comments the code I've added in (1).
The issue is that when in (3) I choose:
(a) Branch if eligible is Not Equal to 1 then End of Survey = I'm out of the survey no matter my DOB (older or younger than 15)
(b) Branch if eligible is Equal to 1 then End of Survey = I'm not screened out of the survey, no matter my DOB (older or younger than 15)
So it looks like the Embedded data attributed is always "1", which I don't understand.
The issue is that when in (3) I choose:
(a) Branch if eligible is Not Equal to 1 then End of Survey = I'm out of the survey no matter my DOB (older or younger than 15)
(b) Branch if eligible is Equal to 1 then End of Survey = I'm not screened out of the survey, no matter my DOB (older or younger than 15)
So it looks like the Embedded data attributed is always "1", which I don't understand.
Hi, is there anyway this question can be done by Qualtrics? I am not familiar with JS. I tried to import the question from the library as in the first comment but then content validation is not available. Anyone know the easy way just to ask year born and qualifies age from there?
Thanks for simplifying the solution @TomG. I've read your's and others' threads on moment.js and I think I have it set up right, but I must be missing something. Do I need to assign an embedded data field labeled "age" (I don't know how to do this.
Here is what I've done so far, in the header:
<script src="https://cdnjs.cloudflare.com/ajax/libs/moment.js/2.21.0/moment.min.js"></script>
And this in the question JS field:
Qualtrics.SurveyEngine.addOnload(function()
{
/*Place your JavaScript here to run when the page loads*/
});
Qualtrics.SurveyEngine.addOnPageSubmit(function() {
var age = moment().diff(moment(jQuery("#"+this.questionId+" .InputText").val()), 'years');
Qualtrics.SurveyEngine.setEmbeddedData('age', age);
if(age > 13 && age < 25) Qualtrics.SurveyEngine.setEmbeddedData('eligible', '1');
});
Qualtrics.SurveyEngine.addOnUnload(function()
{
/*Place your JavaScript here to run when the page is unloaded*/
});
Thanks for your expertise!
> @TomG said:
> I don't remember seeing this question before @mjs5az resurrected it, but the answers given are doing it the hard way and the accepted answer isn't always accurate. You can do everything @nkumich asked for by using moment.js and adding this script to the DOB question:
> ```
> Qualtrics.SurveyEngine.addOnPageSubmit(function() {
> var age = moment().diff(moment(jQuery("#"+this.questionId+" .InputText").val()), 'years');
> Qualtrics.SurveyEngine.setEmbeddedData('age', age);
> if(age > 13 && age < 25) Qualtrics.SurveyEngine.setEmbeddedData('eligible', '1');
> });
> ```
> Demo survey
Here is what I've done so far, in the header:
<script src="https://cdnjs.cloudflare.com/ajax/libs/moment.js/2.21.0/moment.min.js"></script>
And this in the question JS field:
Qualtrics.SurveyEngine.addOnload(function()
{
/*Place your JavaScript here to run when the page loads*/
});
Qualtrics.SurveyEngine.addOnPageSubmit(function() {
var age = moment().diff(moment(jQuery("#"+this.questionId+" .InputText").val()), 'years');
Qualtrics.SurveyEngine.setEmbeddedData('age', age);
if(age > 13 && age < 25) Qualtrics.SurveyEngine.setEmbeddedData('eligible', '1');
});
Qualtrics.SurveyEngine.addOnUnload(function()
{
/*Place your JavaScript here to run when the page is unloaded*/
});
Thanks for your expertise!
> @TomG said:
> I don't remember seeing this question before @mjs5az resurrected it, but the answers given are doing it the hard way and the accepted answer isn't always accurate. You can do everything @nkumich asked for by using moment.js and adding this script to the DOB question:
> ```
> Qualtrics.SurveyEngine.addOnPageSubmit(function() {
> var age = moment().diff(moment(jQuery("#"+this.questionId+" .InputText").val()), 'years');
> Qualtrics.SurveyEngine.setEmbeddedData('age', age);
> if(age > 13 && age < 25) Qualtrics.SurveyEngine.setEmbeddedData('eligible', '1');
> });
> ```
> Demo survey
> @nkumich said:
> Thanks for simplifying the solution @TomG. I've read your's and others' threads on moment.js and I think I have it set up right, but I must be missing something. Do I need to assign an embedded data field labeled "age" (I don't know how to do this.
You don't need to do anything additional with "age" for it to work. However, if you want age saved in your response data, you need to initialize it in your survey flow before the block with the DOB question.
If it is not working, make sure you added the moment.js script to your survey header.
> Thanks for simplifying the solution @TomG. I've read your's and others' threads on moment.js and I think I have it set up right, but I must be missing something. Do I need to assign an embedded data field labeled "age" (I don't know how to do this.
You don't need to do anything additional with "age" for it to work. However, if you want age saved in your response data, you need to initialize it in your survey flow before the block with the DOB question.
If it is not working, make sure you added the moment.js script to your survey header.
Hmm, I checked the header, and it's good. It's still not validating. Should I be replacing any of the JS with my own code, e.g. ("#"+this.questionId+" .InputText")? Inserting the questionID?
Leave a Reply
Enter your E-mail address. We'll send you an e-mail with instructions to reset your password.